write a program to add the diagonal of two-dimensional array.
Answer
Diagonal are the position where value of x axis and y axis cordinates are same. For example.
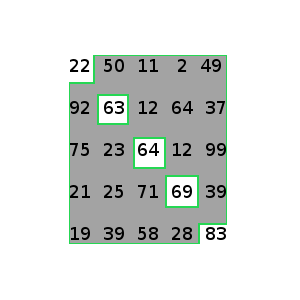
using System; namespace Add_Diagonal { public class Program { public static void Main(string[] args) { int[,] num= { {22,50,11, 2, 49}, {92,63,12,64,37}, {75,23,64,12,99}, {21,25,71,69,39}, {19,39,58,28,83}}; //Getting Row and Column Length int rowlength = num.GetLength(0); int columnlength = num.GetLength(1); int total=0; Console.Write("Diagonals are : "); for(int row=0; row<rowlength; row++) { for(int column=0; column<columnlength; column++) { if(row==column) { Console.Write(num[row,column] + " "); total += num[row,column]; } } } Console.WriteLine(": Sum : " + total); } } }Output
Diagonals are : 22 63 64 69 83 : Sum : 301
_