- What is multi dimensional array?
- How to declare and initialize multi dimensional array in C#?
- How to use multi dimensional array in C# programming?
The multi-dimensional array in C# is such type of array that contains more than one row to store data on it. The multi-dimensional array is also known as a rectangular array in c sharp because it has the same length of each row. It can be a two-dimensional array or three-dimensional array or more. It contains more than one comma (,) within single rectangular brackets (“[ , , ,]”). To storing and accessing the elements from a multidimensional array, you need to use a nested loop in the program. The following example will help you to figure out the concept of a multidimensional array.
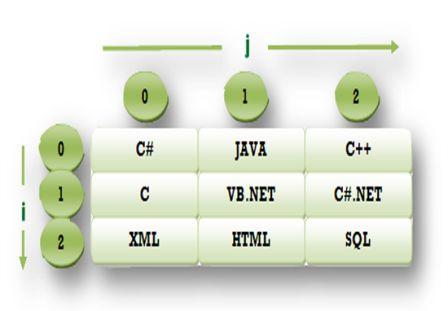
Programming Example of multidimensional array in C#:
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace multi_dimensional_array { class Program { static void Main(string[] args) { int i, j; //Declaring multi dimensional array string[,] Books = new string[3, 3]; for (i = 0; i < 3; i++) { for (j = 0; j < 3; j++) { Console.Write("\nEnter Book Name for {0}. Row and {1}. column:\t", i + 1, j + 1); Books[i, j] = Console.ReadLine(); } } Console.WriteLine("\n\n========================="); Console.WriteLine("All the element of Books array is:\n\n"); //Formatting Output Console.Write("\t1\t2\t3\n\n"); //outer loop for accessing rows for (i = 0; i < 3; i++) { Console.Write("{0}.\t", i + 1); //inner or nested loop for accessing column of each row for (j = 0; j < 3; j++) { Console.Write("{0}\t", Books[i, j]); } Console.Write("\n"); } Console.WriteLine("\n\n========================="); Console.ReadLine(); } } }
Output
Enter Book Name for 1. Row and 1. column: C#
Enter Book Name for 1. Row and 1. column: JAVA
Enter Book Name for 1. Row and 1. column: C++
Enter Book Name for 1. Row and 1. column: C
Enter Book Name for 1. Row and 1. column: VB.NET
Enter Book Name for 1. Row and 1. column: C#.NET
Enter Book Name for 1. Row and 1. column: XML
Enter Book Name for 1. Row and 1. column: HTML
Enter Book Name for 1. Row and 1. column: SQL
===============================================
All the element of Books array is:
1 2 3
1. C# JAVA C++
2. C VB.NET C#.NET
3. XML HTML SQL
__
In the preceding example, we create a two-dimensional array named Books which size is [3,3]. It means, this array has three rows and each row contains three columns. Each row can be accessed using an outer loop and each column of rows can be accessed using a nested loop inside the outer loop as follow.
for (i = 0; i < 3; i++) //outer loop for accessing rows { Console.Write("{0}.\t", i + 1); //inner or nested loop for accessing column of each row for (j = 0; j < 3; j++) { Console.Write("{0}\t", Books[i,j]); } Console.Write("\n"); }
Summary
In this chapter you learned about multi dimensional array in C#. You also learned how to use it in c sharp programming. In next chapter you will learn about param array in C#.