In this chapter you will learn
- What is Generic Stack<T> in C#?
- How to Initialize Generic Stack<T> in C#?
- Programming Example
What is Generic Stack<T> in C#?
The stack is based on Last In First Out [LIFO] mechanism. It is required when you need to access recently added item first. For example, all of you would have used Undo function. When you press Ctrl + Z then the last changes made by you reversed. It is an example of a stack.
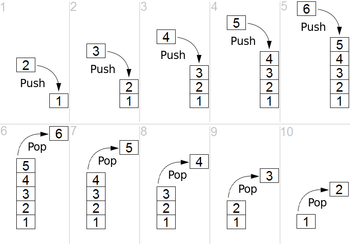
How to initialize Stack<T>?
Stack<string> mystack=new Stack<string>();
Important Properties and Methods of Stack
Properties | Description |
---|---|
Count |
Returns the total count of elements in the Stack. |
Methods | Description |
---|---|
Push |
Inserts an item at the top of the stack. |
Peek |
Returns the top item from the stack. |
Pop |
Removes and returns items from the top of the stack. |
Contains |
Checks whether an item exists in the stack or not. |
Clear |
Removes all items from the stack. |
Programming Example
using System; using System.Collections.Generic; namespace GenericStackExample { public class Program { public static void Main(string[] args) { Stack<string> mystack=new Stack<string>(); //Adding Item in stack mystack.Push("Sunday"); mystack.Push("Monday"); mystack.Push("Tuesday"); mystack.Push("Wednesday"); mystack.Push("Thursday"); mystack.Push("Friday"); mystack.Push("Saturday"); print(mystack); //Accessing Top Item without removing it. Console.WriteLine("\nTop Item is : {0}",mystack.Peek()); //Removing Item from Stack Console.WriteLine("\nRemoved Top Item of Stack : " + mystack.Pop()); Console.WriteLine("\nNow Stack's Items are : "); print(mystack); } public static void print(Stack<string> sp) { foreach(string s in sp) { Console.Write(s.ToString() + " | "); } } } }
Output
Saturday | Friday | Thursday | Wednesday | Tuesday | Monday | Sunday |
Top Item is : Saturday
Removed Top Item of Stack : Saturday
Now Stack's Items are :
Friday | Thursday | Wednesday | Tuesday | Monday | Sunday |
_
Summary
In this tutorial you learned about Generic Stack<T> In C# with programming example.