1. How to add Controllers in ASP.NET MVC 5 Project?
2. How to add a View Page for Controllers in MVC 5?
In the previous chapter, you learned basics of Controller. However, the theory is not as important as practical is. In this chapter, I have added a very simple controller and their view page to make you understand how controller and view page works together.
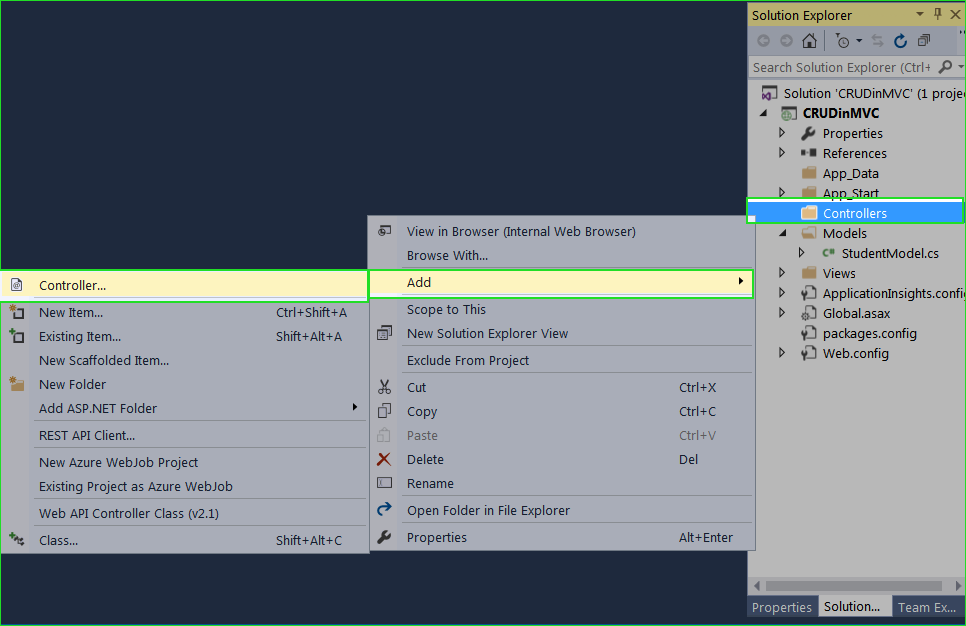
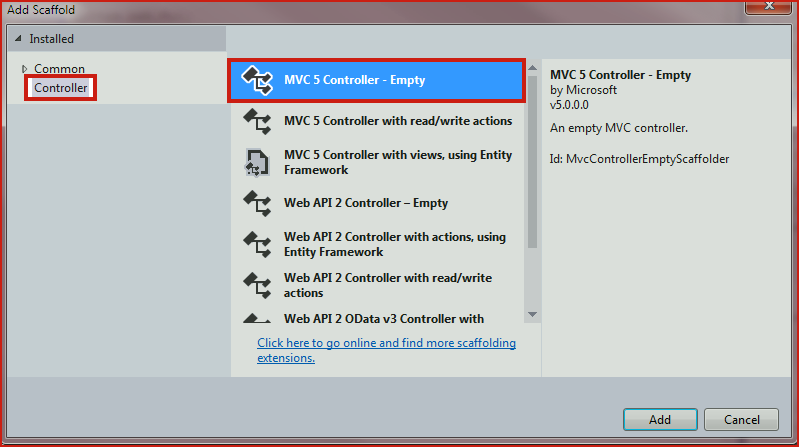
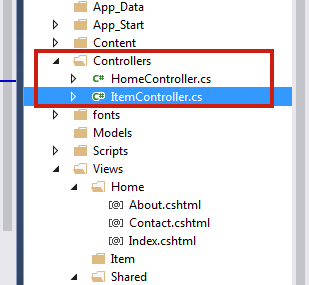
Code
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; namespace CompShop.Controllers { public class ItemController : Controller { // GET: Item public ActionResult Index() { return View(); } } }Explanation:
This controller will execute when user browse following url:
http://localhost:1233/Item/index
In the above link, Item is controller
and Index is action method
.
When user click on the link, it will search for ItemController
with Index()
Action method. Controllers keeps action method that gets executed when user needs them. There must be a ViewPage Index.cshtml
in Item Folder, otherwise you will get error message.
Adding a ViewPage for Index method
Index
Action Method and select Add View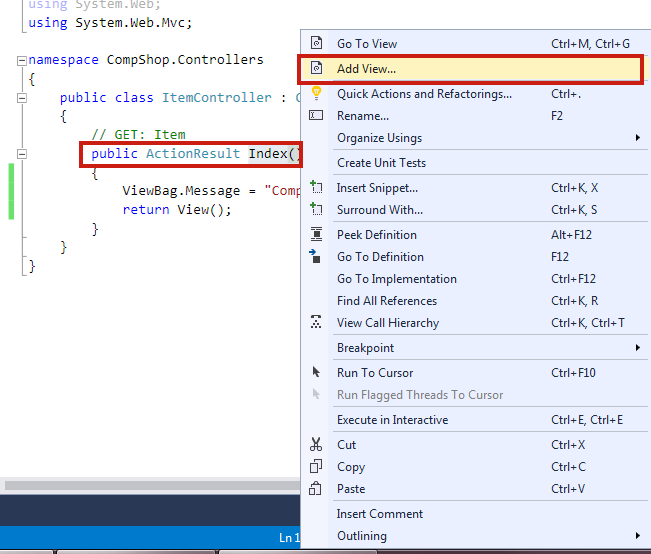
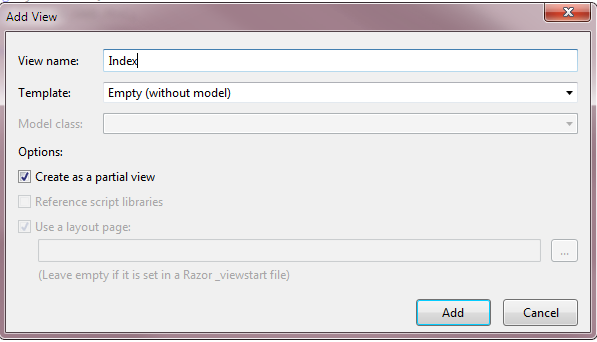
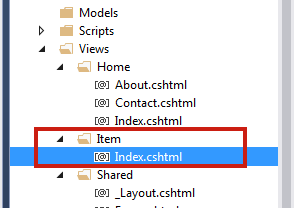
1. Add following codes in
ItemController.cs
file.
using System.Web.Mvc; namespace CompShop.Controllers { public class ItemController : Controller { // GET: Item public ActionResult Index() { ViewBag.ItemList = "Computer Shop Item List Page"; return View(); } } }2. Add Following code in Item
Index.cshtml
file.
<h1>@ViewBag.ItemList</h1>
3. Press F5 to run your project and go to following link:
http://localhost:1233/Item/Index
4. Output
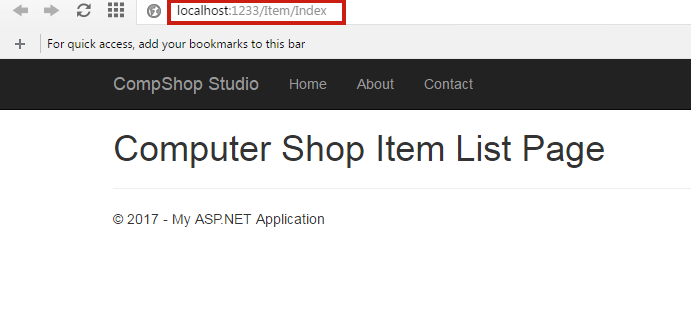
Summary
In this chapter, you seen that there is an ActionResult Index()
method. You must be thinking about ActionResult. In this next chapter, we will learn all ActionResult method with the help of programming example.