1. How to create, select, rename and delete sql database using ado.net c#
Most of the times you need to create SQL Database programmatically. With C# ADO.Net you can easily do it.
Create a Database using ADO.NET C#
Here, I am going to create ComputerShop database programmatically. You can use this code in windows application or web application or even console application.
Programming Exampleusing System; using System.Data.SqlClient; namespace CreateDatabase { class Program { static void Main(string[] args) { SqlConnection con = new SqlConnection(@"Data Source=.\SQLEXPRESS;Initial Catalog=master;Integrated Security=True"); string query = "Create Database ComputerShop"; SqlCommand cmd = new SqlCommand(query, con); try { con.Open(); cmd.ExecuteNonQuery(); Console.WriteLine("Database Created Successfully"); } catch(SqlException e) { Console.WriteLine("Error Generated. Details: " + e.ToString()); } finally { con.Close(); Console.ReadKey(); } } } }
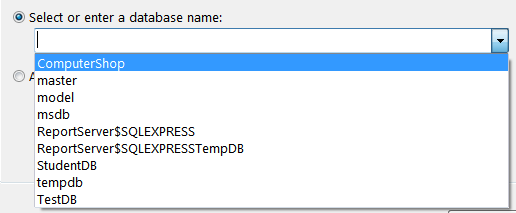
Rename a Database using ADO.NET C#
You can rename a database using ado.net c# like this.
using System; using System.Data.SqlClient; namespace RenameDatabase { class Program { static void Main(string[] args) { SqlConnection con = new SqlConnection(@"Data Source=.\SQLEXPRESS;Initial Catalog=master;Integrated Security=True"); string query = "ALTER DATABASE ComputerShop MODIFY NAME = MobileShop"; SqlCommand cmd = new SqlCommand(query, con); try { con.Open(); cmd.ExecuteNonQuery(); Console.WriteLine("Database Renamed Successfully"); } catch(SqlException e) { Console.WriteLine("Error Generated. Details: " + e.ToString()); } finally { con.Close(); Console.ReadKey(); } } } }
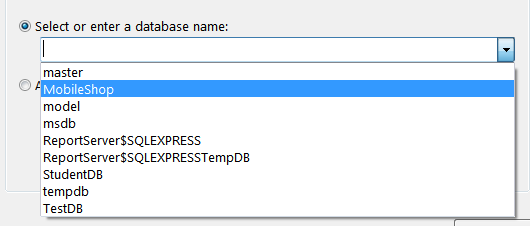
Select a Database using ADO.NET C#
You can select SQL Database in ado.net by passing database name in the connection string.
SqlConnection con = new SqlConnection(@"Data Source=.\SQLEXPRESS;Initial Catalog=MobileShop;Integrated Security=True");
Drop or Delete a Database using ADO.NET C#
In order to delete a database using ado.net run the following block of code.
using System; using System.Data.SqlClient; namespace DeleteDatabase { class Program { static void Main(string[] args) { SqlConnection con = new SqlConnection(@"Data Source=.\SQLEXPRESS;Initial Catalog=master;Integrated Security=True"); string query = "DROP DATABASE ComputerShop"; SqlCommand cmd = new SqlCommand(query, con); try { con.Open(); cmd.ExecuteNonQuery(); Console.WriteLine("Database Deleted Successfully"); } catch(SqlException e) { Console.WriteLine("Error Generated. Details: " + e.ToString()); } finally { con.Close(); Console.ReadKey(); } } } }
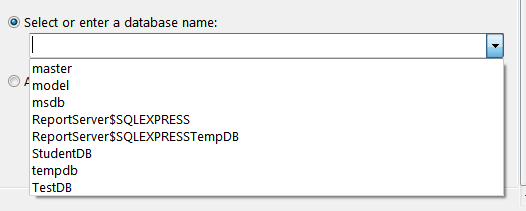
Summary
In this chapter, you learned how to create, select, rename and delete SQL database using ADO.NET C#. I have given the complete programming example and you can use this code in your web application as well as a windows application. In the next chapter, you will learn to Create, Update, Rename and Delete SQL Table using ADO.NET C#.